Mastering the Essentials: Exploring the Depths of Your JavaScript Skills
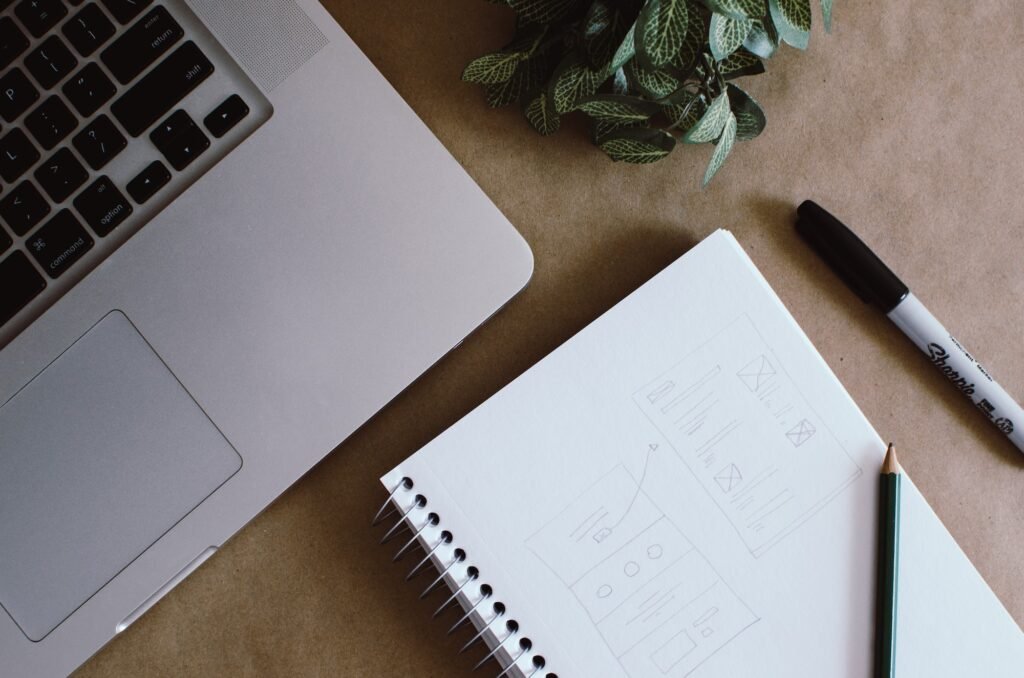
Welcome to our cheery and informative blog post on "Mastering the Essentials: Exploring the Depths of Your JavaScript Skills"! Whether you’re a seasoned web developer or just starting your journey in the world of coding, this comprehensive guide will help you take your JavaScript skills to the next level. We’ll dive deep into the fundamentals, cover advanced concepts, and provide plenty of practical examples and exercises along the way. So, grab your favorite beverage, sit back, and let’s embark on this exciting coding adventure together!
Table of Contents
- Introduction to JavaScript
- Variables and Data Types
- Control Flow and Loops
- Functions and Scope
- Arrays and Objects
- DOM Manipulation
- Asynchronous JavaScript
- ES6 and Beyond
- 8.1 Arrow Functions
- 8.2 Template Literals
- Frequently Asked Questions (FAQ)
- Conclusion
Now, let’s get started with the basics of JavaScript!
Introduction to JavaScript
JavaScript, often abbreviated as JS, is a high-level, interpreted programming language that allows you to add interactivity and dynamic behavior to your websites. It was created by Brendan Eich in 1995 and quickly became one of the most popular programming languages in the world. JavaScript is primarily used for client-side web development, but it has also gained popularity in server-side and mobile app development.
One of the key strengths of JavaScript is its versatility. It provides developers with the power to manipulate web page elements, handle user interactions, perform calculations, and make network requests, among many other things. JavaScript code can be embedded directly into HTML files or included as separate external files.
Variables and Data Types
Before we dive into the depths of JavaScript, let’s start by understanding how variables and data types work in this language.
Declaring Variables
In JavaScript, variables are containers that store data. To declare a variable, you can use the var
, let
, or const
keyword, followed by the variable name. For example:
var myVariable;
let anotherVariable = 42;
const pi = 3.14159;
The var
keyword was traditionally used to declare variables in JavaScript, but with the introduction of ES6, let
and const
have become the preferred options. While let
allows you to declare variables that can be reassigned, const
is used for declaring constants that cannot be changed once assigned.
Working with Data Types
JavaScript supports several data types, including:
- Primitive types:
number
,string
,boolean
,null
,undefined
, andsymbol
. - Reference types:
object
, which includes arrays, functions, and objects.
Understanding and working with different data types is crucial for effective JavaScript programming. For example, you can perform mathematical calculations using numbers, manipulate text with strings, or make logical decisions using booleans.
Control Flow and Loops
In this section, we’ll explore the control flow and looping structures in JavaScript, which allow you to control the flow of execution in your code and repeat certain tasks.
Conditional Statements
Conditional statements, such as if
, else if
, and else
, enable you to execute different blocks of code based on certain conditions. For instance:
let age = 18;
if (age >= 18) {
console.log("You are eligible to vote!");
} else {
console.log("Sorry, you must be at least 18 years old to vote.");
}
In the above example, the code checks if the age
variable is greater than or equal to 18. If it is, the first block of code is executed; otherwise, the second block is executed.
Looping Structures
Loops allow you to repeat a block of code multiple times. JavaScript provides several looping structures, including for
, while
, and do...while
.
The for
loop is commonly used when you know the exact number of iterations you need. Here’s an example:
for (let i = 0; i < 5; i++) {
console.log("Iteration: " + i);
}
In the above code snippet, the loop will execute five times, and the value of i
will be incremented by 1 after each iteration.
The while
loop is useful when you want to repeat a block of code until a certain condition is met. Here's an example:
let count = 0;
while (count < 5) {
console.log("Count: " + count);
count++;
}
The do...while
loop is similar to the while
loop, but it guarantees that the block of code is executed at least once, even if the condition is initially false.
Stay tuned as we continue exploring more advanced concepts and techniques in JavaScript. Remember to practice and experiment with code examples to enhance your understanding and master the essentials of this powerful programming language.
Conclusion
Congratulations on making it to the end of this comprehensive guide on mastering the essentials of JavaScript! We've covered a wide range of topics, from the basics of variables and data types to advanced concepts like asynchronous JavaScript and ES6 features.
By now, you should have a solid foundation in JavaScript and be ready to tackle more complex projects. Remember to keep practicing, experimenting, and exploring new possibilities. The more you code and challenge yourself, the better you'll become.
JavaScript is an exciting and dynamic language that continues to evolve. Stay up to date with the latest developments and best practices in the JavaScript ecosystem. Explore libraries, frameworks, and tools that can enhance your productivity and help you build amazing web applications.
Thank you for joining us on this coding adventure! We hope you found this blog post informative, engaging, and useful. Happy coding!
Frequently Asked Questions (FAQ)
Q: What is JavaScript?
A: JavaScript is a high-level, interpreted programming language that enables web developers to add interactivity and dynamic behavior to websites.
Q: How do I declare a variable in JavaScript?
A: You can declare a variable using the var
, let
, or const
keyword, followed by the variable name.
Q: What are the different looping structures in JavaScript?
A: JavaScript provides for
, while
, and do...while
loops for repeating blocks of code.
Q: Can I use JavaScript on the server-side?
A: Yes, with the introduction of Node.js, JavaScript can now be used for server-side development as well.
Q: What is the latest version of JavaScript?
A: The latest version of JavaScript is ECMAScript 2022, also known as ES12.
Q: Are there any resources to practice JavaScript coding?
A: Yes, there are several online platforms, coding challenges, and tutorials available to practice and improve your JavaScript skills.
Q: Can I build mobile apps using JavaScript?
A: Yes, JavaScript frameworks like React Native and Ionic allow you to build cross-platform mobile apps using JavaScript.
Q: Is JavaScript the same as Java?
A: No, JavaScript and Java are two different programming languages with distinct syntax and use cases.
Q: Are there any JavaScript frameworks or libraries I should learn?
A: Some popular JavaScript frameworks and libraries include React, Angular, Vue.js, and jQuery. It's beneficial to learn at least one of them for modern web development.
Q: Can I make network requests using JavaScript?
A: Yes, JavaScript provides features like AJAX and the Fetch API to make HTTP requests to external APIs.
Q: Can I manipulate web page elements using JavaScript?
A: Yes, using the Document Object Model (DOM), JavaScript can dynamically modify HTML elements, styles, and content.
Q: Is JavaScript case-sensitive?
A: Yes, JavaScript is case-sensitive, so myVar
and myvar
are considered as two different variables.
Q: Are there any coding conventions for JavaScript?
A: Yes, there are widely accepted coding conventions like the Airbnb JavaScript Style Guide or the Google JavaScript Style Guide that help maintain consistency and readability in code.
Q: Where can I find more JavaScript resources and documentation?
A: The Mozilla Developer Network (MDN) website is an excellent resource for JavaScript documentation, tutorials, and guides.
Q: Can I use JavaScript to create games?
A: Yes, JavaScript is widely used for game development, and there are libraries like Phaser and PixiJS specifically designed for creating games.
Q: Is JavaScript only used for web development?
A: While JavaScript is primarily used for web development, it can also be used for server-side development, mobile app development, desktop app development, and even IoT (Internet of Things) projects.
Q: How can I debug JavaScript code?
A: Most modern web browsers come with built-in developer tools that include a JavaScript console for debugging. You can also use tools like Visual Studio Code with integrated debugging capabilities.
Q: Can I use JavaScript to create desktop applications?
A: Yes, with frameworks like Electron, you can build desktop applications using JavaScript, HTML, and CSS.
Q: Is JavaScript backward compatible?
A: Yes, JavaScript is designed to be backward compatible, meaning that code written in older versions should still work in newer environments.
Q: What is the benefit of using a JavaScript framework?
A: JavaScript frameworks provide a structured and efficient way to develop web applications, with built-in features, optimizations, and community support.
Q: How can I improve my JavaScript coding skills?
A: Practice regularly, read code written by others, participate in coding challenges, and explore open-source projects. Additionally, attending JavaScript conferences and joining developer communities can help you grow as a JavaScript developer.